目录
一、什么是自动装配
1.按名称自动装配(autowire=”byName”)
[2. 不使用自动装配(autowire=”no”)](#2. 不使用自动装配(autowire=”no”))
[3 .按类型自动装配(autowire=”byType”)](#3 .按类型自动装配(autowire=”byType”))
[4. 构造函数自动装配(autowire=”constructor”)](#4. 构造函数自动装配(autowire=”constructor”))
二、使用注解进行Bean自动装配
@Resource
@Autowired
@Qualifier
三.自动装配的优缺点
一、什么是自动装配
自动装配就是指 Spring 容器在不使用 和 标签的情况下,可以自动装配(autowire)相互协作的 Bean 之间的关联关系,将一个 Bean 注入其他 Bean 的 Property 中。
自动装配需要配置 元素的 autowire 属性。
具体的属性如下表:
名称 |
说明 |
no |
默认值,表示不使用自动装配,Bean 依赖必须通过 ref 元素定义。 |
byName |
根据 Property 的 name 自动装配,如果一个 Bean 的 name 和另一个 Bean 中的 Property 的 name 相同,则自动装配这个 Bean 到 Property 中。 |
byType |
根据 Property 的数据类型(Type)自动装配,如果一个 Bean 的数据类型兼容另一个 Bean 中 Property 的数据类型,则自动装配。 |
constructor |
类似于 byType,根据构造方法参数的数据类型,进行 byType 模式的自动装配。 |
autodetect(3.0版本不支持) |
如果 Bean 中有默认的构造方法,则用 constructor 模式,否则用 byType 模式。 |
自动装配说明(此段说明转自狂神说Spring04:自动装配)
- 自动装配是使用spring满足bean依赖的一种方法
- spring会在应用上下文中为某个bean寻找其依赖的bean。
Spring中bean有三种装配机制,分别是:
- 在xml中显式配置;
- 在java中显式配置(注解);
- 隐式的bean发现机制和自动装配。
这里我们主要讲第三种:自动化的装配bean。
Spring的自动装配需要从两个角度来实现,或者说是两个操作:
- 组件扫描(component scanning):spring会自动发现应用上下文中所创建的bean;
- 自动装配(autowiring):spring自动满足bean之间的依赖,也就是我们说的IoC/DI;
组件扫描和自动装配组合发挥巨大威力,使得显示的配置降低到最少
这里讲得比较全面
下面举例子:
创建一个Person类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| public class Person { private Man man; public Person() { System.out.println("在Person的构造函数内"); } public Person(Man man) { System.out.println("在Person的有参构造函数内"); this.man = man; } public void man() { man.show(); } public Man getMan() { return man; } public void setMan(Man man) { this.man = man; } }
|

创建一个Man类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| public class Man { private String name; private int age; public Man() { System.out.println("在man的构造函数内"); } public Man(String name, int age) { System.out.println("在man的有参构造函数内"); this.name = name; this.age = age; } public void show() { System.out.println("名称:" + name + "\n年龄:" + age); } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
|

1.按名称自动装配(autowire=”byName”)
1 2 3 4 5 6 7 8 9 10 11 12
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id="man" class="com.pojo.Man"> <constructor-arg value="pojo" /> <constructor-arg value="12" type="int" /> </bean> <bean id="person" class="com.pojo.Person" autowire="byName"> </bean> </beans>
|

Test测试类
1 2 3 4 5
| public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("Beans2.xml"); Person person = (Person) context.getBean("person"); person.man(); }
|

结果如下
在man的有参构造函数内
在Person的构造函数内
名称:pojo
年龄:12
小结:
当一个bean节点带有 autowire byName的属性时。
- 将查找其类中所有的set方法名,例如setCat,获得将set去掉并且首字母小写的字符串,即cat。
- 去spring容器中寻找是否有此字符串名称id的对象。
- 如果有,就取出注入;如果没有,就报空指针异常。
2. 不使用自动装配(autowire=”no”)
更改xml的配置如下
1 2 3 4 5 6 7 8 9 10 11 12 13
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id="man" class="com.pojo.Man"> <constructor-arg value="pojo" /> <constructor-arg value="12" type="int" /> </bean> <bean id="person" class="com.pojo.Person" autowire="no"> <constructor-arg ref="man" type="com.pojo.Man"/> </bean> </beans>
|

得到结果
在man的有参构造函数内
在Person的有参构造函数内
名称:pojo
年龄:12
3 .按类型自动装配(autowire=”byType”)
更改xml
1 2 3 4 5
| <bean id="man1" class="com.pojo.Man"> <constructor-arg value="pojo" /> <constructor-arg value="12" type="int" /> </bean> <bean id="person" class="com.pojo.Person" autowire="byType"/>
|

运行结果:
在man的有参构造函数内
在Person的构造函数内
名称:bianchengbang
年龄:12
如果您有相同类型的多个 Bean,则注入失败,并且引发异常
4. 构造函数自动装配(autowire=”constructor”)
1 2 3 4 5
| <bean id="man" class="com.pojo.Man"> <constructor-arg value="pojo" /> <constructor-arg value="12" type="int" /> </bean> <bean id="person" class="com.pojoPerson" autowire="constructor"/>
|

运行结果:
在man的有参构造函数内
在Person的有参构造函数内
名称:bianchengbang
年龄:12
二、使用注解进行Bean自动装配
使用注解
jdk1.5开始支持注解,spring2.5开始全面支持注解。
准备工作:利用注解的方式注入属性。
Spring 中常用的注解如下。不了解的可以先看看这个
Spring-使用注解开发-万元课程的课上笔记_man_one的博客-CSDN博客
创建两个动物类:
1 2 3 4 5
| public class Cat { public void shout(){ System.out.println("我是修(>^ω^<)喵"); } }
|

1 2 3 4 5
| public class Dog { public void shout(){ System.out.println("我是修~(@^_^@)~狗"); } }
|

创建主人People类(重要):
@Resource
- @Resource如有指定的name属性,先按该属性进行byName方式查找装配;
- 其次再进行默认的byName方式进行装配;
- 如果以上都不成功,则按byType的方式自动装配。
- 都不成功,则报异常。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| import javax.annotation.Resource;
public class People {
@Resource(name = "cat2") private Cat cat;
@Resource private Dog dog; private String name;
public Cat getCat() { return cat; }
public Dog getDog() { return dog; }
public String getName() { return name; }
@Override public String toString() { return "People{" + "cat=" + cat + ", dog=" + dog + ", name='" + name + '\'' + '}'; }
}
|

配置xml
Spring 默认不使用注解装配 Bean,因此需要在配置文件中添加 context:annotation-config/,启用注解。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/aop https://www.springframework.org/schema/aop/spring-aop.xsd">
<context:annotation-config/>
<bean id="cat1" class="com.kuang.pojo.Cat"/> <bean id="cat2" class="com.kuang.pojo.Cat"/> <bean id="dog132" class="com.kuang.pojo.Dog"/> <bean id="people" class="com.kuang.pojo.People"/>
</beans>
|

不要忘了配置maven坐标
1 2 3 4 5 6 7 8
| @Test public void test1(){ ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml");
People people = context.getBean("people", People.class); people.getDog().shout(); people.getCat().shout(); }
|
测试结果
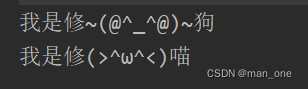

@Autowired
- @Autowired是按类型自动转配的,不支持id匹配。
- 需要导入 spring-aop的包!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| public class User { @Autowired private Cat cat; @Autowired private Dog dog; private String str;
public Cat getCat() { return cat; } public Dog getDog() { return dog; } public String getStr() { return str; } }
|

@Autowired(required=false) 说明:false,对象可以为null;true,对象必须存对象,不能为null。
1 2 3
| //如果允许对象为null,设置required = false,默认为true @Autowired(required = false) private Cat cat
|
1、将User类中的set方法去掉,使用@Autowired注解
@Qualifier
- @Autowired是根据类型自动装配的,加上@Qualifier则可以根据byName的方式自动装配
- @Qualifier不能单独使用
1 2 3 4 5 6
| @Autowired @Qualifier(value = "cat2") private Cat cat; @Autowired @Qualifier(value = "dog2") private Dog dog;
|

测试输出成功!
三.自动装配的优缺点
优点
缺点
- 不能自动装配简单数据类型,比如 int、boolean、String 等。
- 相比较显示装配,自动装配不受程序员控制。